Interviewing tips for software engineers applying to Zalando. Before Amazon, I worked for Zalando for over 2 years. During that time I interviewed countless developers, from junior to tech leads, mostly in the Android space. In this post, I share what I was looking for and how to best prepare for the software engineer interviews. Continue reading
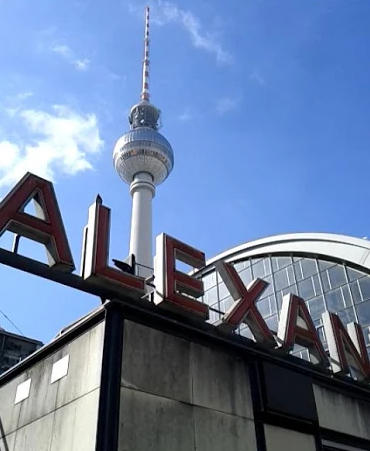